Highlight Returning Customers in WooCommerce Orders Dashboard
Returning customers are a good indicator of how much your customer enjoyed their experience and would like to buy from you again. Since they are the backbone of any business, they need special attention when handling their orders.
In this article, I will show how you to mark the returning customers directly in the WooCommerce orders list. Happily, WordPress is highly customizable and with a simple code snippet, you can extend the orders’ interface and detect returning customers based on their email address or ID.
As shown below, the goal is to change the background color of orders from returning customers to green.
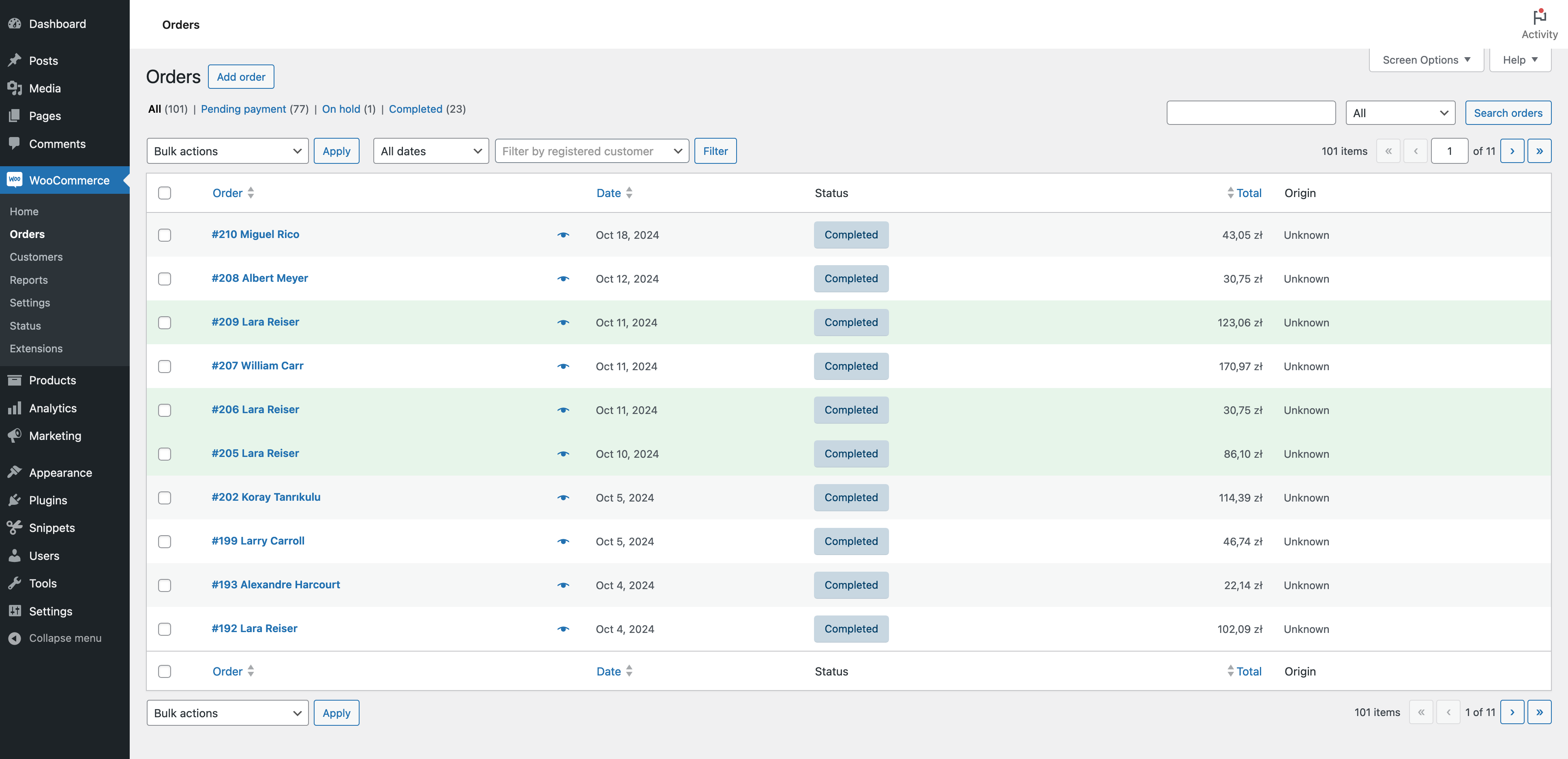
Introduction
The Problem with WooCommerce Checkout
WooCommerce stores use various checkout options which can make it difficult to determine which customers are new and which are returning.
Simply speaking, they can complete the orders in one of four different ways:
- By registering an account and being logged in.
- By using a duplicate user account with the same email address.
- By having an account but choosing not to log in, purchasing as a guest.
- By not registering at all, only providing an email during checkout as a guest.
WooCommerce can technically handle each case as if it were an order from four different customers. Therefore, the snippet we are going to create cannot depend only on user accounts to determine whether the customer is returning.
Fortunately, WooCommerce, like other plugins, allows for some customization through additional hooks. The following snippet can extend the built-in WooCommerce order dashboard to identify returning customers.
What is the Code Snippet?
A code snippet is a small block of code that can modify your WordPress site without requiring a complete plugin. It is the most efficient and simplest way to extend WooCommerce as well as other plugins.
Solution
It does not matter how customers choose to complete check out - as a guest or logged-in user - the code snippet will recognize them in each scenario.
In the next section, we will show you how to implement this feature step-by-step. Once implemented, this snippet will flag returning customers directly in the WooCommerce admin order list.
To make it working, you need to use both parts of the code within the same snippet.
Step 1. Find the Returning Customer by ID or Email Address
The following code checks the customer’s ID and email address for each order displayed in the admin dashboard. If it matches any existing customer in your database (whether they registered an account or not), the customer will be automatically marked as “returning”.
function bis_is_returning_customer( $order ) {
if ( is_numeric( $order ) ) {
$order = wc_get_order( $order );
} else if ( ! is_a( $order, 'WC_Order' ) ) {
return;
}
$order_id = $order->get_id();
$order_email = $order->get_billing_email();
$customer_id = $order->get_customer_id();
$order_date = $order->get_date_created();
// Store the info as a custom field
$is_returning_customer = get_post_meta( $order_id, 'is_returning_customer', true );
if ( ! metadata_exists( 'post', $order_id, 'is_returning_customer' ) ) {
// Check by email & ID
$is_new_customer_email = bis_check_if_new_customer( $order_email, $order_id, $order_date );
$is_new_customer_id = ( $customer_id ) ? bis_check_if_new_customer( $customer_id, $order_id, $order_date ) : true;
$is_returning_customer = ( $is_new_customer_email && $is_new_customer_id ) ? 0 : 1;
update_post_meta( $order_id, 'is_returning_customer', $is_returning_customer );
}
return $is_returning_customer;
}
function bis_check_if_new_customer( $in, $exclude_order = null, $until_date = null, $return_order = false ) {
// Excluded ids should be an array
if ( ! empty( $exclude_order ) ) {
$exclude_order = ( is_array( $exclude_order ) ) ? $exclude_order : array( $exclude_order );
}
// Get most recent orders
if ( is_numeric( $in ) ) {
$args = array(
'customer_id' => $in,
'status' => array( 'completed', 'pending' ),
'exclude' => $exclude_order,
);
} else {
$args = array(
'billing_email' => $in,
'status' => array( 'completed', 'pending' ),
'exclude' => $exclude_order,
);
}
// Limit to date
if ( ! empty( $until_date ) ) {
$args['date_created'] = '<' . strtotime( $until_date );
}
$args['orderby'] = 'date';
$args['order'] = 'DESC';
$customer_orders = wc_get_orders( $args );
// Count number of orders
$count = count( $customer_orders );
// Return "true" when customer has already one order
if ( $return_order ) {
return ( $customer_orders && ! empty( $customer_orders[0] ) ) ? $customer_orders[0] : false;
} else {
return ( $count > 0 ) ? false : true;
}
}
Step 2. Add Inline CSS to Order Dashboard
After identifying a returning customer, you can make their orders stand out visually in your admin dashboard. Here is the next part of the code that can conditionally highlight these orders.
function bis_add_returning_customer_class( $classes, $order ) {
$is_returning_customer = bis_is_returning_customer( $order );
if ( $is_returning_customer ) {
$classes[] = 'returning-customer';
}
return $classes;
}
add_filter( "woocommerce_shop_order_list_table_order_css_classes", 'bis_add_returning_customer_class', 10, 2 );
function bis_add_order_admin_css() {
global $current_screen;
if ( empty( $current_screen->post_type ) || $current_screen->post_type !== 'shop_order' || ! function_exists( 'wc_get_order_types' ) ) {
return;
}
echo '<style>
.returning-customer {
background-color: #e7f5ea !important;
}
</style>';
}
add_action( 'admin_head', 'bis_add_order_admin_css' );